Initialization
Trackswitch requires jQuery and Fontawesome to be included to work, e.g.
<!-- ... -->
<link href="https://maxcdn.bootstrapcdn.com/font-awesome/4.7.0/css/font-awesome.min.css" rel="stylesheet" integrity="sha384-wvfXpqpZZVQGK6TAh5PVlGOfQNHSoD2xbE+QkPxCAFlNEevoEH3Sl0sibVcOQVnN" crossorigin="anonymous" />
<link rel="stylesheet" href="trackswitch.min.css" />
<!-- ... -->
<div class="player">
<p>
Example trackswitch.js instance.
</p>
<img src="mix.png" class="seekable"/>
<ts-track title="Drums" data-img="drums.png">
<ts-source src="drums.mp3" type="audio/mpeg"></ts-source>
</ts-track>
<ts-track title="Synth" data-img="synth.png">
<ts-source src="synth.mp3" type="audio/mpeg"></ts-source>
</ts-track>
<ts-track title="Bass" data-img="bass.png">
<ts-source src="bass.mp3" type="audio/mpeg"></ts-source>
</ts-track>
<ts-track title="Violins" data-img="violins.png">
<ts-source src="violins.mp3" type="audio/mpeg"></ts-source>
</ts-track>
</div>
<!-- ... -->
<script src="https://code.jquery.com/jquery-3.2.1.min.js" integrity="sha256-hwg4gsxgFZhOsEEamdOYGBf13FyQuiTwlAQgxVSNgt4="crossorigin="anonymous"></script>
<script src="trackswitch.min.js"></script>
<script type="text/javascript">
jQuery(document).ready(function() {
jQuery(".player").trackSwitch({spacebar: true});
});
</script>
<!-- ... -->
Alternatively you can of course use Browserify.
Configuration
Tracks
Each track is contained in one ts-track
element and must contain one or more ts-source
elements:
<div class="player">
<ts-track title="Violins">
<ts-source src="violins.mp3"></ts-source>
</ts-track>
<ts-track title="Synth">
<ts-source src="synth.mp3"></ts-source>
</ts-track>
<ts-track title="Bass">
<ts-source src="bass.mp3"></ts-source>
</ts-track>
<ts-track title="Drums">
<ts-source src="drums.mp3"></ts-source>
</ts-track>
</div>
Note that each ts-source
should always contain a closing element.
Fallback Audio Files
Due to a messy Browser compatibility situation it is recommended you define multiple ts-source
s with different formats for each ts-track
.
It is recommended, but not required, that you to define the MIME type in each ts-source
.
<div class="player">
<ts-track title="Violins">
<ts-source src="violins.mp3" type="audio/mpeg"></ts-source>
<ts-source src="violins.mp4" type="audio/mp4"></ts-source>
</ts-track>
<ts-track title="Synth">
<ts-source src="synth.mp3" type="audio/mpeg"></ts-source>
<ts-source src="synth.mp4" type="audio/mp4"></ts-source>
</ts-track>
<ts-track title="Bass">
<ts-source src="bass.mp3" type="audio/mpeg"></ts-source>
<ts-source src="bass.mp4" type="audio/mp4"></ts-source>
</ts-track>
<ts-track title="Drums">
<ts-source src="drums.mp3" type="audio/mpeg"></ts-source>
<ts-source src="drums.mp4" type="audio/mp4"></ts-source>
</ts-track>
</div>
Track Styling
You can use CSS to style each individual ts-track
element:
<div class="player">
<ts-track title="Violins" style="background-color: #156090;">
<ts-source src="violins.mp3"></ts-source>
</ts-track>
<ts-track title="Synth" style="background-color: #15737D;">
<ts-source src="synth.mp3"></ts-source>
</ts-track>
<ts-track title="Bass" style="background-color: #158769;">
<ts-source src="bass.mp3"></ts-source>
</ts-track>
<ts-track title="Drums" style="background-color: #159858;">
<ts-source src="drums.mp3"></ts-source>
</ts-track>
</div>
Solo Tracks
You can preselect solo for individual tracks by using the solo
attribute within the ts-track
element, like this: <ts-track title="Violins" solo>
.
<div class="player">
<ts-track title="Violins" solo>
<ts-source src="violins.mp3" type="audio/mpeg"></ts-source>
</ts-track>
<ts-track title="Synth" solo>
<ts-source src="synth.mp3" type="audio/mpeg"></ts-source>
</ts-track>
<ts-track title="Bass">
<ts-source src="bass.mp3" type="audio/mpeg"></ts-source>
</ts-track>
<ts-track title="Drums">
<ts-source src="drums.mp3" type="audio/mpeg"></ts-source>
</ts-track>
</div>
Mute Tracks
You can preselect mute for individual tracks by using the mute
attribute within the ts-track
element, like this: <ts-track title="Bass" mute>
.
<div class="player">
<ts-track title="Violins">
<ts-source src="violins.mp3" type="audio/mpeg"></ts-source>
</ts-track>
<ts-track title="Synth">
<ts-source src="synth.mp3" type="audio/mpeg"></ts-source>
</ts-track>
<ts-track title="Bass" mute>
<ts-source src="bass.mp3" type="audio/mpeg"></ts-source>
</ts-track>
<ts-track title="Drums" mute>
<ts-source src="drums.mp3" type="audio/mpeg"></ts-source>
</ts-track>
</div>
Player Behaviour
The player allows for several different settings to be enabled or disabled. This is done using a settings object, for example:
var settings = {
onlyradiosolo: true,
repeat: true,
};
$(".player").trackSwitch(settings);
Any combination of the following boolean flags is possible, where the indicated value depicts the default value.
mute
: Iftrue
show mute buttons. Defaults totrue
.solo
: Iftrue
show solo buttons. Defaults totrue
.globalsolo
: Iftrue
mute all other trackswitch instances when playback starts. Defaults totrue
.repeat
: Iftrue
initialize player with repeat button enabled. Defaults tofalse
.radiosolo
: Iftrue
allow only 1 track to be soloed at a time (makes the shift+click behaviour the default). Useful for comparing rather than mixing tracks. Defaults tofalse
.onlyradiosolo
: Iftrue
sets bothmute: false
andradiosolo: true
in one argument. Useful for one track at a time comparison. Also makes the whole track row clickable. Defaults tofalse
.spacebar
: Iftrue
bind the spacebar key to play/pause. Can be turned on for more than one player, but only affect the most recently activated. Defaults tofalse
.tabview
: Iftrue
change the layout so tracks are arranged in a ‘tab view’. This saves vertical space, for example on a presentation. Defaults tofalse
.
Additional Player Elements
You can add aditional elements directly into the player, e.g. a paragraph <p>
with some custom styling.
<div class="player">
<p style="text-align: center;">Example with padded and centered text.</p>
<ts-track title="Violins">
<ts-source src="violins.mp3"></ts-source>
</ts-track>
<ts-track title="Synth">
<ts-source src="synth.mp3"></ts-source>
</ts-track>
<ts-track title="Bass">
<ts-source src="bass.mp3"></ts-source>
</ts-track>
<ts-track title="Drums">
<ts-source src="drums.mp3"></ts-source>
</ts-track>
</div>
Example with padded and centered text.
Additional and Seekable Player Image
You can include images related to the audio content, which can optionally act as a seekable play-head area (similar to the SoundCloud player for example). In the example below, the player below will contain two images, the first of which will also act as a seekable player. Any number of the images can be set, but only one seekable image is acceptable.
<div class="player">
<img class="seekable" src="mix.png">
<img src="cover.jpg">
<ts-track title="Violins">
<ts-source src="violins.mp3"></ts-source>
</ts-track>
<ts-track title="Synth">
<ts-source src="synth.mp3"></ts-source>
</ts-track>
<ts-track title="Bass">
<ts-source src="bass.mp3"></ts-source>
</ts-track>
<ts-track title="Drums">
<ts-source src="drums.mp3"></ts-source>
</ts-track>
</div>
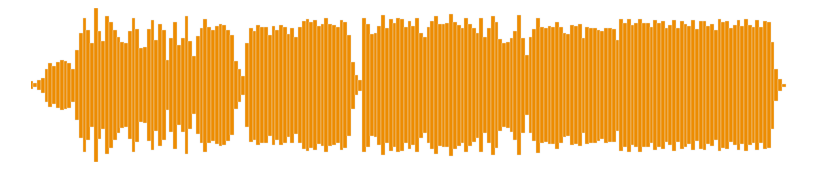
Seekable Image Start/Stop Margin
As you can see, the start end end times of the plot don’t exactly match with the seekhead. In this situation you can specify the seekable area margin for each seekable image.
This can be done by specifying the start and stop points as a percentage of the image using the data-seek-margin-left
and data-seek-margin-right
attributes.
<div class="player">
<img class="seekable" data-seek-margin-left="4" data-seek-margin-right="4" src="mix.png">
<ts-track title="Violins" data-img="violins.png">
<ts-source src="violins.mp3"></ts-source>
</ts-track>
<ts-track title="Synth" data-img="synth.png">
<ts-source src="synth.mp3"></ts-source>
</ts-track>
<ts-track title="Bass" data-img="bass.png">
<ts-source src="bass.mp3"></ts-source>
</ts-track>
<ts-track title="Drums" data-img="drums.png">
<ts-source src="drums.mp3"></ts-source>
</ts-track>
</div>
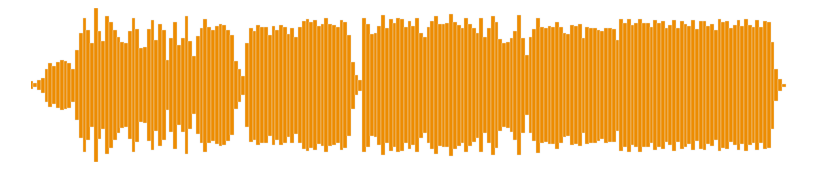
Seekable Image For Each Track
You can optionally define a more specific image to replace the default when a particular track is played back in solo. This is done by adding an image link in the data-img
attribute of the chosen ts-track
element, as seen below.
<div class="player">
<img class="seekable" data-seek-margin-left="4" data-seek-margin-right="4" src="mix.png">
<ts-track title="Violins" data-img="violins.png">
<ts-source src="violins.mp3"></ts-source>
</ts-track>
<ts-track title="Synth" data-img="synth.png">
<ts-source src="synth.mp3"></ts-source>
</ts-track>
<ts-track title="Bass" data-img="bass.png">
<ts-source src="bass.mp3"></ts-source>
</ts-track>
<ts-track title="Drums" data-img="drums.png">
<ts-source src="drums.mp3"></ts-source>
</ts-track>
</div>
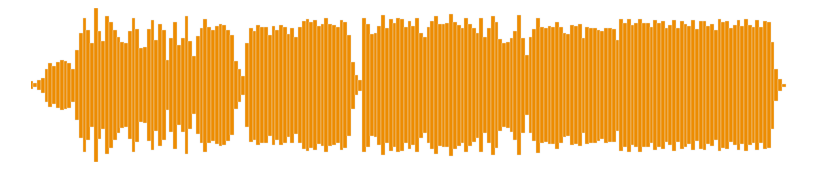
In the example above, there is a default image as well as specific images defined for the track.
You do not need to define a specific image for every track. If there is no image defined for a track when it is soloed, the default image will be used.
Seekable Image Styling
The images can be positioned using normal CSS (eg, width
and margin
properties). For non-seekable images, this style can be applied using the style
attribute.
For seekable
images this style must be defined in a ‘data-style’ properly rather than the usual ‘style’ property.
<div class="player">
<img style="margin: 20px auto;" src="cover.jpg">
<img data-style="width: 80%; margin: auto;" class="seekable" data-seek-margin-left="4" data-seek-margin-right="4" src="mix.png">
<ts-track title="Violins" data-img="violins.png">
<ts-source src="violins.mp3"></ts-source>
</ts-track>
<ts-track title="Synth" data-img="synth.png">
<ts-source src="synth.mp3"></ts-source>
</ts-track>
<ts-track title="Bass" data-img="bass.png">
<ts-source src="bass.mp3"></ts-source>
</ts-track>
<ts-track title="Drums" data-img="drums.png">
<ts-source src="drums.mp3"></ts-source>
</ts-track>
</div>
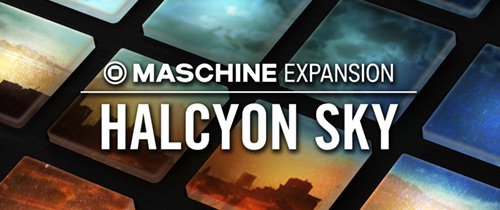
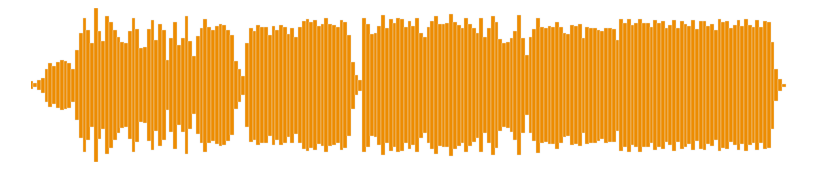